반응형
문제
You are given an integer array height
of length n
. There are n
vertical lines drawn such that the two endpoints of the ith
line are (i, 0)
and (i, height[i])
.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return the maximum amount of water a container can store.
Notice that you may not slant the container.
Example 1:
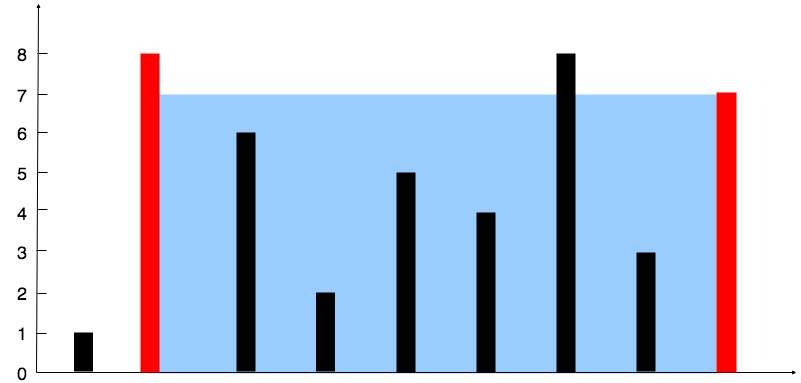
Input: height = [1,8,6,2,5,4,8,3,7]
Output: 49
Explanation: The above vertical lines are represented by array [1,8,6,2,5,4,8,3,7]. In this case, the max area of water (blue section) the container can contain is 49.
Example 2:
Input: height = [1,1]
Output: 1
Constraints:
n == height.length
2 <= n <= 105
0 <= height[i] <= 104
https://leetcode.com/problems/container-with-most-water
풀이
while문을 사용하여 양쪽에서 물 양을 비교하며 최대값만 저장하게끔 진행했다.
어차피 양쪽의 높이 중 낮은 높이에 따라 양이 결정되니, 이중 for문을 이용하여 전부 다 비교하기 보다는 높은 쪽을 고정해 두고 낮은 쪽만 움직이게끔 하는 것이 낫다고 생각했다.
코드
class Solution {
public int maxArea(int[] height) {
int max = 0;
int leftIdx = 0;
int rightIdx = height.length - 1;
while(leftIdx < rightIdx) {
int water = (rightIdx - leftIdx) * Math.min(height[leftIdx], height[rightIdx]);
max = Math.max(max, water);
if(height[leftIdx] < height[rightIdx]) {
leftIdx++;
} else {
rightIdx--;
}
}
return max;
}
}
결과
Runtime : 5 ms
Memory Usage : 57.90 MB
반응형
'LeetCode' 카테고리의 다른 글
13. Roman to Integer (2) | 2024.12.20 |
---|---|
12. Integer to Roman (3) | 2024.11.30 |
10. Regular Expression Matching (0) | 2024.11.18 |
8. String to Integer (atoi) (1) | 2024.11.12 |
7. Reverse Integer (1) | 2024.11.10 |